일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- useState
- SSR
- bitcoin
- node.js
- built in object
- nextJS
- API
- solidity
- error
- CSS
- middleware
- Redux
- typeScript
- CLASS
- JavaScript
- Interface
- Props
- tailwindcss
- hardhat
- express.js
- 기준
- evm
- HTML
- Ethereum
- graphQL
- blockchain
- web
- REACT
- concept
- 삶
- Today
- Total
ReasonJun
Redux : middleware / Thunk 본문
middleware
is a way to extend Redux with custom functionality. It is a function that sits between the action being dispatched and the action reaching the reducers. It allows you to intercept actions and do things like logging, routing, or asynchronous actions.
Middleware is a composable function, which means that you can combine multiple middleware together. This makes it easy to add new functionality to your Redux application without having to rewrite your reducers.
Here are some of the benefits of using middleware in Redux:
- It allows you to add custom functionality to your Redux application without having to rewrite your reducers.
- It is composable, so you can combine multiple middleware together.
- It is easy to test, since middleware functions are just regular JavaScript functions.
Here are some of the examples of middleware in Redux:
- Logging middleware can be used to log all actions that are dispatched to the store. This can be useful for debugging purposes.
- Routing middleware can be used to route actions to different parts of your application. This can be useful for complex applications with multiple views.
- Asynchronous middleware can be used to perform asynchronous tasks, such as making HTTP requests or calling APIs. This can be useful for applications that need to interact with external services.
To use middleware in Redux, you need to use the applyMiddleware() function. This function takes an array of middleware functions as an argument. The middleware functions will then be executed in the order that they are passed to the applyMiddleware() function.
Here is an example of how to use middleware in Redux:
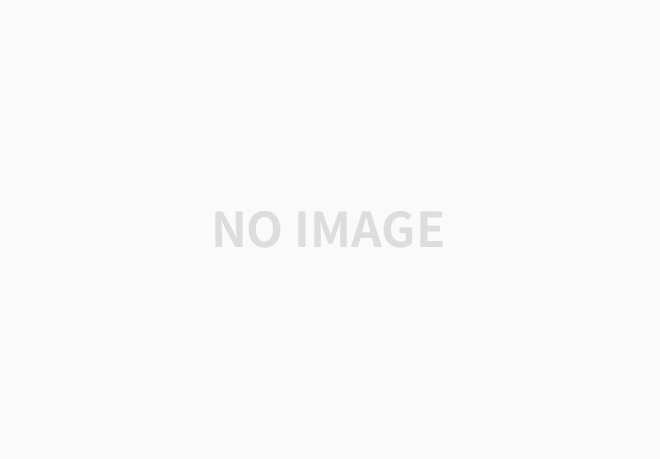
const loggerMiddleware = (store: any) => (next: any) => (action: any) => {
console.log("store", store)
console.log('action', action);
next(action);
}
const middleware = applyMiddleware(thunk,loggerMiddleware);
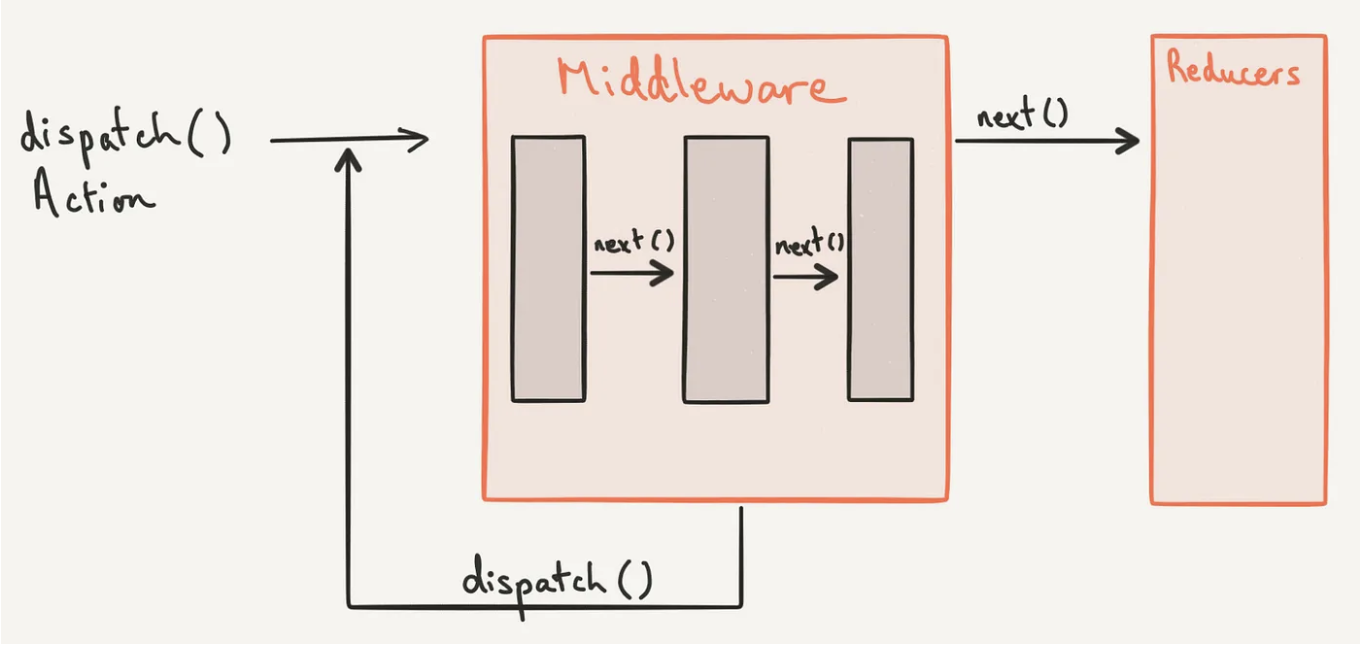
Thunk
is a middleware that allows you to write action creators that return a function instead of an action object. This function can then be used to perform asynchronous tasks, such as making HTTP requests or calling APIs.
Here is an example of how to use Thunk middleware:
import { createStore, applyMiddleware } from 'redux';
import thunk from 'redux-thunk';
const reducer = (state, action) => {
switch (action.type) {
case 'GET_TODOS':
return { todos: ['TODO 1', 'TODO 2'] };
default:
return state;
}
};
const store = createStore(reducer, applyMiddleware(thunk));
const getTodos = () => {
return dispatch => {
// Perform an asynchronous task, such as making an HTTP request
setTimeout(() => {
dispatch({ type: 'GET_TODOS' });
}, 1000);
};
};
const store.dispatch(getTodos());
In this example, the getTodos function is an action creator that returns a function. This function is then passed to the dispatch function. The dispatch function will then call the function, which will perform an asynchronous task, such as making an HTTP request. Once the asynchronous task is finished, the dispatch function will dispatch an action to the store.

'Frontend > Redux' 카테고리의 다른 글
Redux : toolkit API : configureStore() (0) | 2023.08.19 |
---|---|
Redux: toolkit (0) | 2023.08.19 |
Redux : Provider / useSelector / useDispatch (0) | 2023.08.19 |
Redux: CombineReducers (0) | 2023.08.19 |
Redux: strict unidirectional data flow (0) | 2023.08.19 |